This is the first module (Python Basics) for Python for Data Science, a course by IBM's Cognitive Class Labs.
OUTLINE:
- Your First Program
- Types
- Expressions and Variables
- String Operations
Your first program
A statement or expression is an instruction the computer will run or execute
Print statement
Simplest program to write
Value (argument) displayed in parentheses
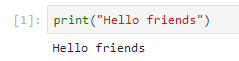
Comment code
Tells others what the code does
# symbol followed by comment
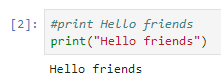
Syntactic error
Python does not understand the code
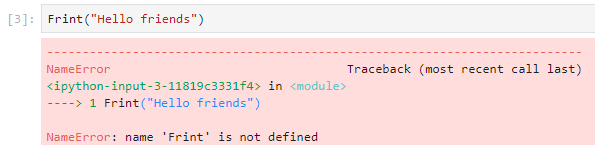
Semantic error
Logic is wrong
Don’t get an error message
Types
Type is how Python represents different types of data
Chart: expression | data type
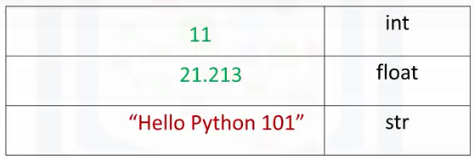
Type command
See the actual data type in Python
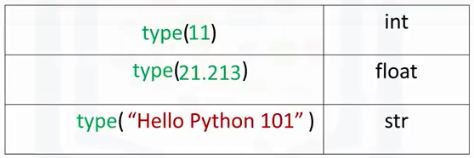
int
Integer
Can be negative or positive

float
“float”
real number
Include integers, but also decimals
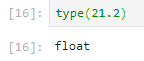
str
“string”
sequence of characters
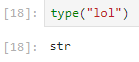
bool
Boolean
T - true
F - false
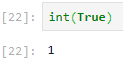
Type-casting
Change the type of expression
Cast int into float:

Cast int into str:

BEWARE: can lose info - Cast float into int:
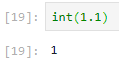
ERROR: Cast str into int when it does not have an integer value:
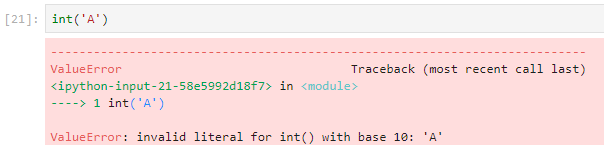
Cast bool into int:
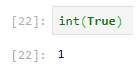
Cast int into bool:
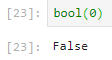
Expressions and Variables
Expression
A type of operation
Basic arithmetic operations
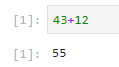
Operand - numbers
Operator - math symbols
//

Double slash
Integer division, the result is rounded
Remember PEMDAS? Ahh, memories...
Variables
Store values
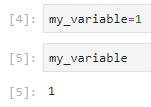
Can change variable
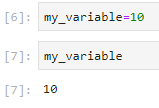
Can store results of expressions

Can perform operations on x and save to a new variable y
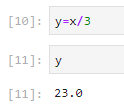
Can use the type command on variables
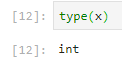
Use meaningful variable names
Can use underscore or capital letter to start a new word
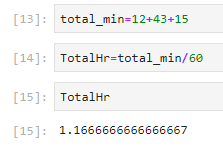
String Operations
Sequence of characters contained within two quotes

A string is an ordered sequence
Each element of the sequence can be accessed using an index represented by the array of numbers

Negative indexing
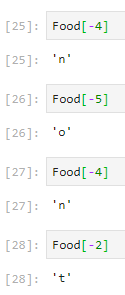
Bind a string to another variable
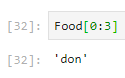
Think of a string as a list or tuple
Collection which is ordered or unchangeable
Treat the string as a sequence and perform sequence operations
Stride
Specifies how many characters to move forward after the first character is retrieved from the string
The third number in the slice command specifies the stride
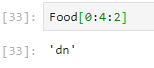
len
Specify the length of the string
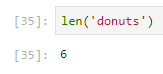
cat
Concatenate
Combine strings using the addition symbols
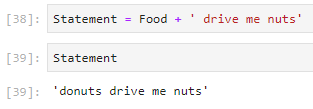
Replicate values of a string
Multiply string
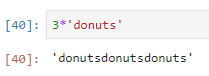
Cannot change value of the string, but can create new string by setting it to the original variable and concatenated with a new string
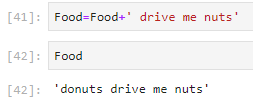
Strings are immutable - they can’t be changed once they have been created
\
Beginning of an escape sequence - represents strings that may be difficult to input
\n
New line
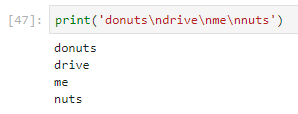
\t
Inserts a ‘tab’
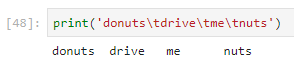
\\
Inserts a ‘\’
Can also insert an ‘r’ in front of the string
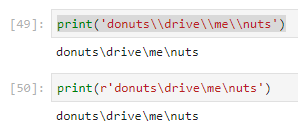
String Methods
Strings are sequences
Have apply methods that work on lists and tuples
Have a second set of methods that just work on strings
upper
Converts lowercase characters to uppercase
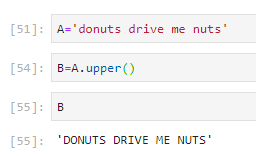
replace
Exchange second argument with the segment
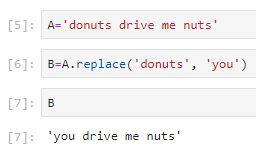
find
Finds sub-strings
Argument is the sub-string you would like to find
Output is -1 if the sub-string is not in the string
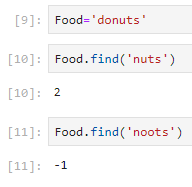
Comments